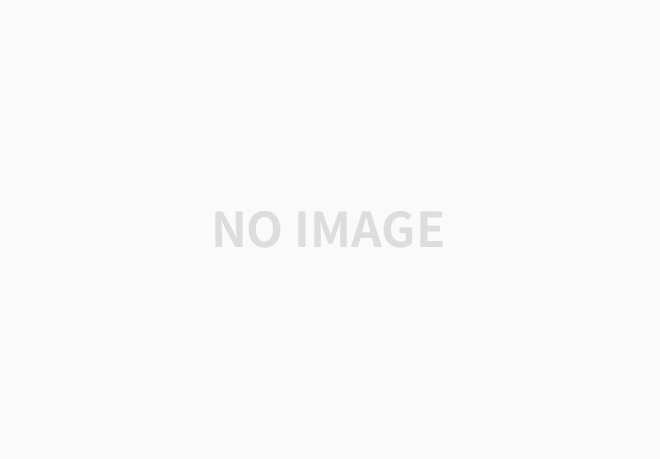
[C#] 실행경로, 시작경로, 현제경로를 가져오는 방법
1. Application.StartupPath
- 현재 application이 존재하는 경로
- winform 인 경우에만 사용가능
- 레지스트리 관계없이 실제 실행 파일
- Application.ExcutablePath 와 동일
- string filePath = Application.StartupPaht + @"testFile.txt";
2. System.Environment.CurrentDirectory
- 현재 작업 실행되는 경로
- winform 이외의 개발 환경에서도 사용가능
- winform 에서는 "C:/Windows/System32" 경로가 나옴 ( svchost.exe 를 통해 서비스가 등록 되기 때문 )
- System.IO.Directory.GetCurrentDirectory() 와 동일
[winform의 경우]
1) Application.StartupPath 를 사용
2) string path = System.Reflection.Assembly.GetExecutingAssembly().Location;
path = System.IO.Path.GetDirectoryName(path);
3. AppDomain.CurrentDomain.BaseDirectory
- 현재 application이 실행되는 경로
- WPF에서 사용
출처: https://rocabilly.tistory.com/114 [프로그램이 좋다]
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace BabyCarrot.Tools
{
public static class Application // 실행 경로 값을 가져오는 프로퍼티 !
{
public static string Root // 프로퍼티
{
get {
string root = AppDomain.CurrentDomain.BaseDirectory; //실행경로 ?
return root;
}
}
}
}
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace BabyCarrot.Tools
{
public class LogManager //
{
private string _path;
//파일 리소스를 사용할때 사용가능한지 확인하고 사용하고 빠르게 놔줘야한다.
//로그를 작성할려면 작성하는 파일을 생성하거나 파일이 있으면 로그를 생성
public LogManager(string path)
{
_path = path;
_SetLogPath();
}
public LogManager() : this(Path.Combine(Application.Root, "Path"))//들어오는 값이 없는 로그의 경우 그냥 경로를 넣어준다? //IO.Path.Combine ->기존 경로에 추가 string변수를 추가해준다는 느낌
{
}
//로그 파일은 하루에 하나씩 생성을 할껀데 현재 이제 로그 메니저가 생성될 당시에 파일 네임을 생성 파일이 없으면 생성 /있으면 추가
private void _SetLogPath()
{
if (!System.IO.Directory.Exists(_path))
{
System.IO.Directory.CreateDirectory(_path);
}
string logFile = DateTime.Now.ToString("yyyyMMdd") + ".txt";
_path = Path.Combine(_path, logFile);
// 원래 기존 경로에다가 logFile 경로를 합쳐준다.
}
public void WriteLine(string data)
{
try
{
using (StreamWriter writer = new StreamWriter(_path, true))
{
writer.WriteLine(DateTime.Now.ToString("yyyyMMdd HH:mm:ss\t") + data);
}
}
catch (Exception ex)
{
throw;
}
}
public void Write(string data)
{
try
{
using (StreamWriter writer = new StreamWriter(_path, true))
{
writer.Write(DateTime.Now.ToString("yyyyMMdd HH:mm:ss\t") + data);
}
}
catch (Exception ex)
{
throw;
}
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using BabyCarrot.Tools; //참조로 연결해줘야한다.
namespace BabyCarrotTest
{
class Program
{
static void Main(string[] args)
{
LogManager log = new LogManager();
log.WriteLine("[Begin Processing]-------");
for (int i = 0; i < 10; i++)
{
log.WriteLine("Processing :" + i);
//Do
System.Threading.Thread.Sleep(500);
log.WriteLine("Done :" + i);
}
log.WriteLine("[End Processing]-------");
}
}
}
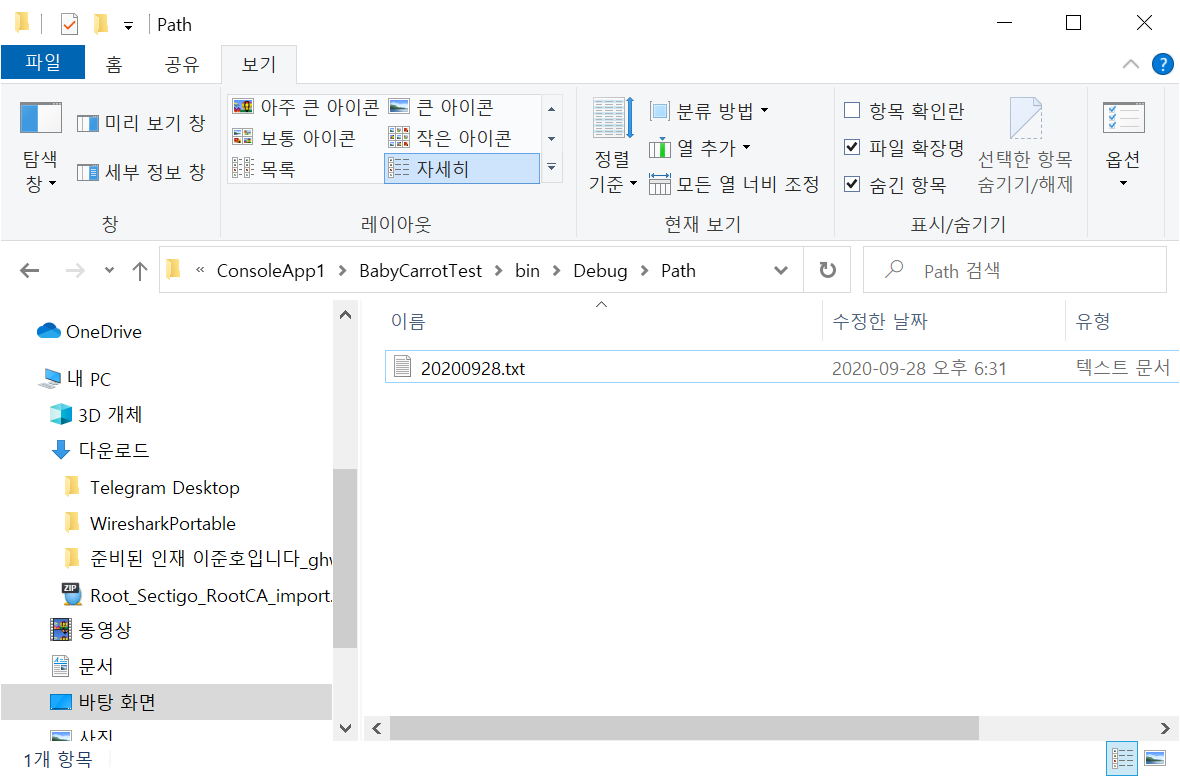
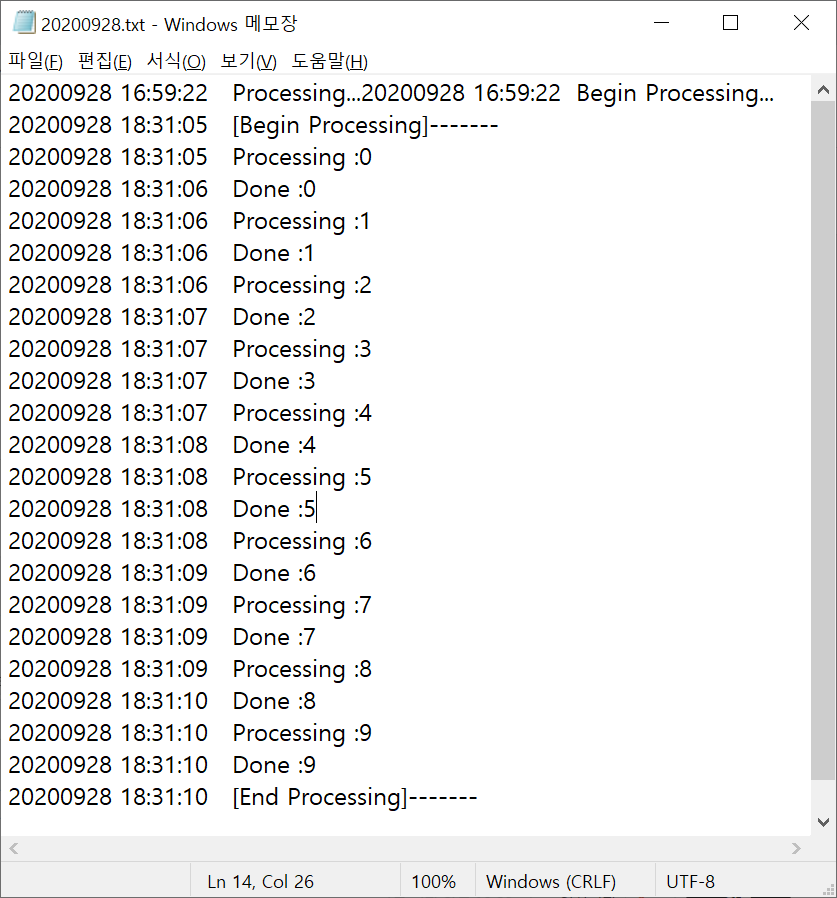
'C#' 카테고리의 다른 글
C# Thread(스레드) 주요 속성과 메서드 ! (1) | 2024.02.08 |
---|---|
EXCEL에서 CODE 128 폰트로 읽을 수 있는 바코드 만들기 렛츠 고우 (18) | 2024.01.22 |
C#- ASP.NET 으로 웹 로그인 화면 만들기(MVC-모델-뷰-컨트롤러) ! (0) | 2024.01.17 |
C# - 네트워크 , Socket , TCP소켓 대해 알아보자 (3) | 2023.10.17 |
C# 문법 - String 문자열을 Enum으로 바꾸는 방법 /Trim(Char[])로 원하는 문자 제거 /구조체의 차이점 복습 (1) | 2023.10.17 |
C# - PDA 프로그램 개발 DataGridView 정렬과 row 이동하기 (DataTable, DataView 사용) (1) | 2023.10.15 |